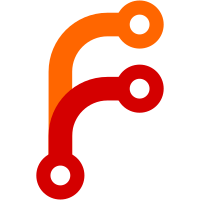
fix usage of `math.random` in various places to provide both a minimum and a maximum number, instead of just maximum. also renamed some files very slightly.
28 lines
542 B
Lua
28 lines
542 B
Lua
function blockgame.get_keys (tab)
|
|
local keyset = {}
|
|
local n = 0
|
|
for key, value in pairs(tab) do
|
|
n = n + 1
|
|
keyset[n] = key
|
|
end
|
|
return keyset
|
|
end
|
|
|
|
function blockgame.shallow_copy_table (tab)
|
|
local result = {}
|
|
for key, value in pairs(tab) do
|
|
result[key] = value
|
|
end
|
|
return result
|
|
end
|
|
|
|
function blockgame.shuffle (tab)
|
|
local keys = blockgame.get_keys(tab)
|
|
local result = {}
|
|
while #keys > 0 do
|
|
local index = math.random(1, #keys)
|
|
local key = table.remove(keys, index)
|
|
table.insert(result, tab[key])
|
|
end
|
|
return result
|
|
end
|